Maps in Dart
5 min read
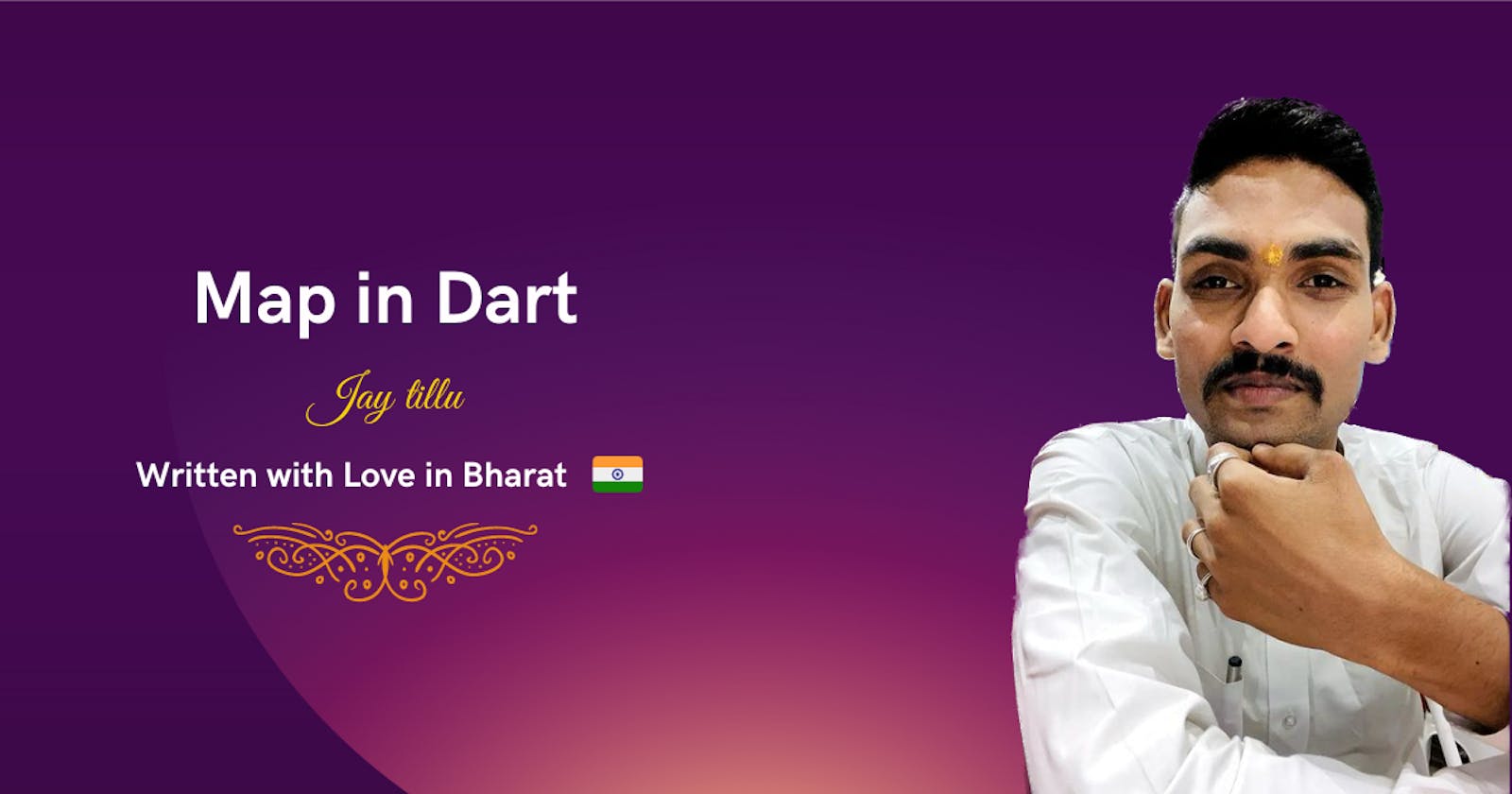
Just like a List, a Map is also a type of collection. In Maps data is stored in key: value pairs. Keys and values can be of any type. The map is a growable collection which means it can shrink and grow at runtime. The map can contain a NULL value as well.
What is a Map in Dart?
A Map is a way to store data where each element has a unique key and an associated value. The key is like a label or an identifier that lets you access its value. Keys must be unique, but values can be repeated.
var ageMap = {
'Alice': 25,
'Bob': 30,
'Charlie': 28,
};
The keys are
Alice
,Bob
, andCharlie
.The values are 25, 30, and 28.
Why Use a Map in Dart?
Maps are great when you need to store information that can be looked up by a specific identifier (key). For example:
Storing the names and scores of players in a game.
Keeping track of items and their prices in a shopping cart.
Saving configurations or settings where you use labels to identify each setting.
Commonly used Map Operations
1. Adding or Updating Values
To add a new key-value pair or update an existing one, you can use square brackets []
with the key.
ageMap['David'] = 32; // Adds 'David' with age 32
ageMap['Alice'] = 26; // Updates 'Alice's age to 26
2. Accessing Values
You can get the value associated with a key by using square brackets []
.
int aliceAge = ageMap['Alice']; // Returns 26
3. Removing Values
To remove a key-value pair, use the remove()
method with the key.
ageMap.remove('Bob'); // Removes 'Bob' and his age
4. Checking if a Key or Value Exists
Use
containsKey()
to check if a key exists.Use
containsValue()
to check if a value exists.
bool hasAlice = ageMap.containsKey('Alice'); // true
bool hasAge30 = ageMap.containsValue(30); // false (since Bob was removed)
Commonly used Map Methods
Here’s a list of important methods you’ll use often when working with Maps in Dart:
1. addAll()
This method adds multiple key-value pairs from another Map to the current one.
var ageMap = {
'Alice': 25,
'Bob': 30,
'Charlie': 28,
};
var newEntries = {
'Eve': 29,
'Frank': 31,
};
ageMap.addAll(newEntries); // Adds 'Eve' and 'Frank' with their ages
2. clear()
The clear()
method removes all the entries from the Map, making it empty.
ageMap.clear(); // Removes all key-value pairs
3. length
The length
property gives you the number of key-value pairs in the Map.
var size = ageMap.length; // Returns the number of entries in the map
4. forEach()
This method allows you to apply a callback function to each key-value pair in the Map.
ageMap.forEach((key, value) {
print('$key is $value years old');
});
5.keys and values
keys
returns all the keys in the Map.values
returns all the values in the Map.Remember this operation returns
Iterable
NOT ARRAY (LIST)You can loop through its values using for loop and forEach. But its not Array.
var allKeys = ageMap.keys; // Returns all keys
var allValues = ageMap.values; // Returns all values
6. isEmpty and isNotEmpty
These are properties that check if the Map has any entries.
bool isEmpty = ageMap.isEmpty; // Returns true if the map has no entries
bool isNotEmpty = ageMap.isNotEmpty; // Returns true if the map has entries
7. update()
The update()
method changes the value for a specific key. If the key doesn’t exist, it throws an error unless you provide a ifAbsent
function.
ageMap.update('Alice', (value) => value + 1); // Increases Alice's age by 1
8. putIfAbsent()
This method adds a key-value pair only if the key is not already in the Map.
ageMap.putIfAbsent('Charlie', () => 28); // Won’t change anything since Charlie already exists
Iterating Over a Map using forEach() and for Loop
Dart makes it easy to loop through the keys and values in a Map. You can use a forEach()
method and for
loop.
ageMap.forEach((key, value) {
print('$key is $value years old');
});
for (var key in ageMap.keys) {
print('Key: $key');
}
for (var value in ageMap.values) {
print('Value: $value');
}
Conclusion
Maps in Dart are incredibly useful for storing key-value pairs and allow you to quickly look up, add, update, and remove data. Whether you’re building a simple app or something more complex, understanding how to work with Maps will make your Dart coding much easier and more efficient.
To summarize, Maps:
Use keys to store and retrieve values.
Provide methods like
addAll()
,remove()
,update()
, and more to manipulate data easily.Offer flexibility by allowing keys and values of any type.
So, guys, That’s it for maps. Feel free to let me know if I miss something. Till then Keep Loving, Keep Coding. And Just like always I’ll catch you up in the next article. 😊
Remember no teacher, no book, no video tutorial, or no blog can teach you everything. As one said Learning is Journey and Journey never ends. Just collect some data from here and there, read it, learn it, practice it, and try to apply it. Don’t feel hesitant about not doing that or not knowing this concept. Remember every programmer was passed from the path on which you are walking right now. Remember Every Master was Once a Beginner. Work hard and Give your best.