Method Overriding in Dart
3 min read
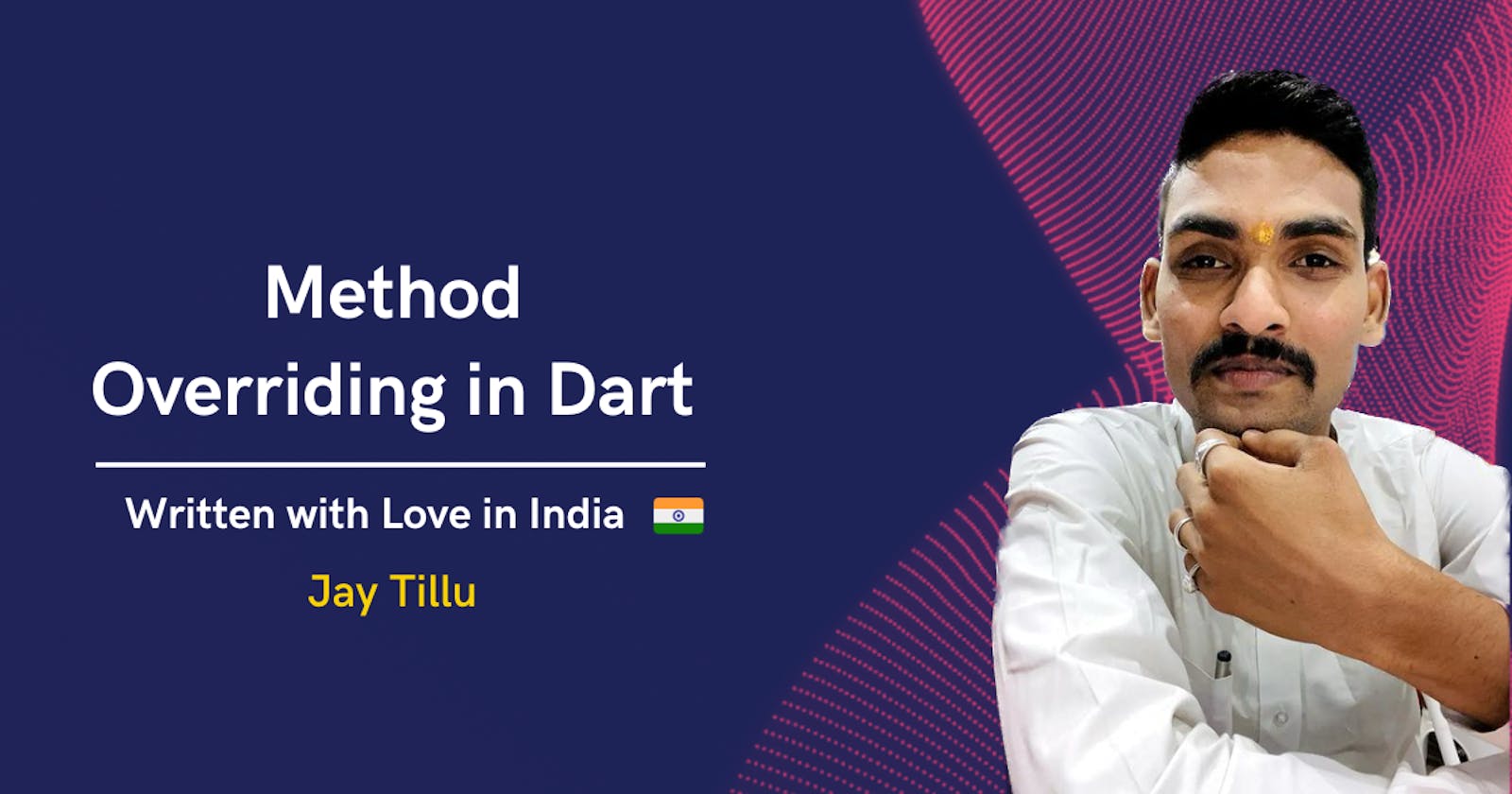
Overriding is done so that a child class can give its implementation to the method that is already provided by the parent class. In this case, the method in the parent class is called the overridden method and the method in the child class is called the overriding method.
The number and data type of the function’s argument must match while overriding the method. The main advantage of method overriding is that the individual class can give its specific implementation to the inherited method without even modifying the parent class code.
So now you don’t need to create the same method again and again in different classes. If you want to put the different implementations in a different class with the same method you can do it by method overriding.
Example
Let’s understand this concept by example. We have three classes here. One parent class is called Car and two child classes are called Jay and Sanket. Parent class has one method named showOwner().
So when showOwner() is called via the parent’s own object then it will have a different owner name. And when showOwner() is called via Jay or Sanket’s object then the same method has a different implementation. The simple example of method overriding… 😉
class Car {
void ShowOwner() => print("This is Parent's car");
}
class Jay extends Car {
@override
void ShowOwner() {
// TODO: implement ShowOwner
print("This JD's car");
}
}
class Sanket extends Car {
@override
void ShowOwner() {
// TODO: implement ShowOwner
print("This is Sanket's car");
}
}
main() {
Car parentCar = new Car();
Jay jdCar = new Jay();
Sanket sanketCar = new Sanket();
parentCar.ShowOwner();
jdCar.ShowOwner();
sanketCar.ShowOwner();
}
Output
This is Parent's car
This JD's car
This is Sanket's car
Conclusion
In Conclusion, Method overriding occurs when a child class redefines a method from its parent class, allowing the child class to provide its own implementation for the method without modifying the parent class code.
Key points covered in the article include:
Definition of Method Overriding: Method overriding is the practice of a child class redefining a method inherited from its parent class.
Purpose of Overriding: It allows the child class to provide its specific implementation for a method without altering the parent class's code.
Requirements for Overriding: The number and data types of the function's arguments in the child class's method must match those in the parent class's method.
Advantages of Method Overriding: It eliminates the need to create the same method multiple times in different classes, as different classes can share a method with unique implementations through overriding.
In summary, method overriding in Dart allows child classes to customize inherited methods, promoting code reusability and flexibility in class hierarchies.