Call Stack in JavaScript - Simplified
3 min read
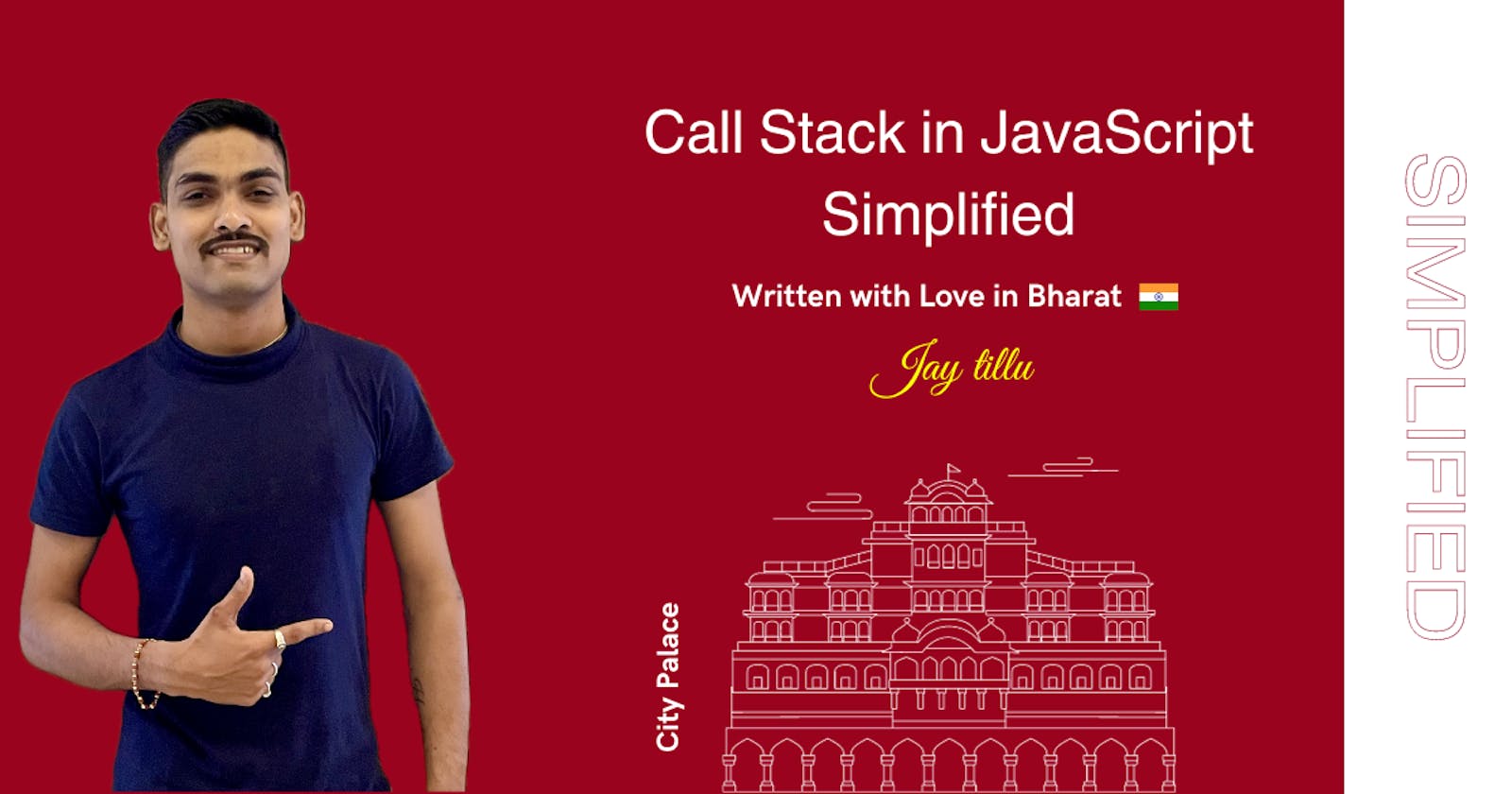
In JavaScript, the call stack is like a to-do list for functions in your program. It follows the rule of "Last In, First Out," meaning the last thing added is the first to be done.
A call stack is like a script's roadmap for a JavaScript Engine. It helps the JavaScript Engine to keep track of which function is currently running and which functions are called from within that function. It's basically a way for the JavaScript Engine to navigate through a script with multiple functions.
Purpose of Call Stack in JavaScript
The call stack in JavaScript has an important job. It keeps track of the order in which functions are called and manages the context of each function's execution. Here's what it does:
Remembering where to go back: When a function is called, the call stack remembers where to go back to when that function is done. It's like noting the page number in a book.
Keeping track of local stuff: Each function has its own set of special things it's using, like variables and information. The call stack keeps track of these so that each function gets what it needs.
Handling repeat tasks: If a function calls itself (which is called recursion), the call stack is crucial. It keeps track of all the times the function is called, like making a list of all the times you play a game.
Managing computer memory: The call stack helps the computer use memory efficiently. It keeps track of which functions are active and which ones are done, so the computer can clean up and use memory wisely.
How Call Stack Works in JavaScript
Here's a simpler breakdown:
Function Calls: When you call a function, it's like adding a task to the top of your to-do list (the call stack). The task includes details about the function, like what it needs to do.
Execution: The code in the function runs step by step. If the function calls another function, that new task is added to the top of the list, and you focus on it.
Return: When a function finishes its job, it's like crossing off the task at the top. Control goes back to the previous task on the list.
function greet(name) {
console.log("Hello, " + name + "!");
}
function welcome() {
console.log("Welcome to the program!");
}
function main() {
let userName = "John";
greet(userName);
welcome();
}
main();
Imagine your to-do list:
main()
is added.greet(userName)
is added (top of the list).greet()
finishes, so it's crossed off.welcome()
is added (new top).welcome()
finishes, so it's crossed off.
Understanding the call stack helps you follow your program's steps. If you have too many tasks, it's like having too much on your to-do list, leading to a "stack overflow" error. In simple terms, the call stack is like the brain's checklist, making sure everything is in order and organized while the computer is working on tasks in a program.