Datatypes in JavaScript
5 min read
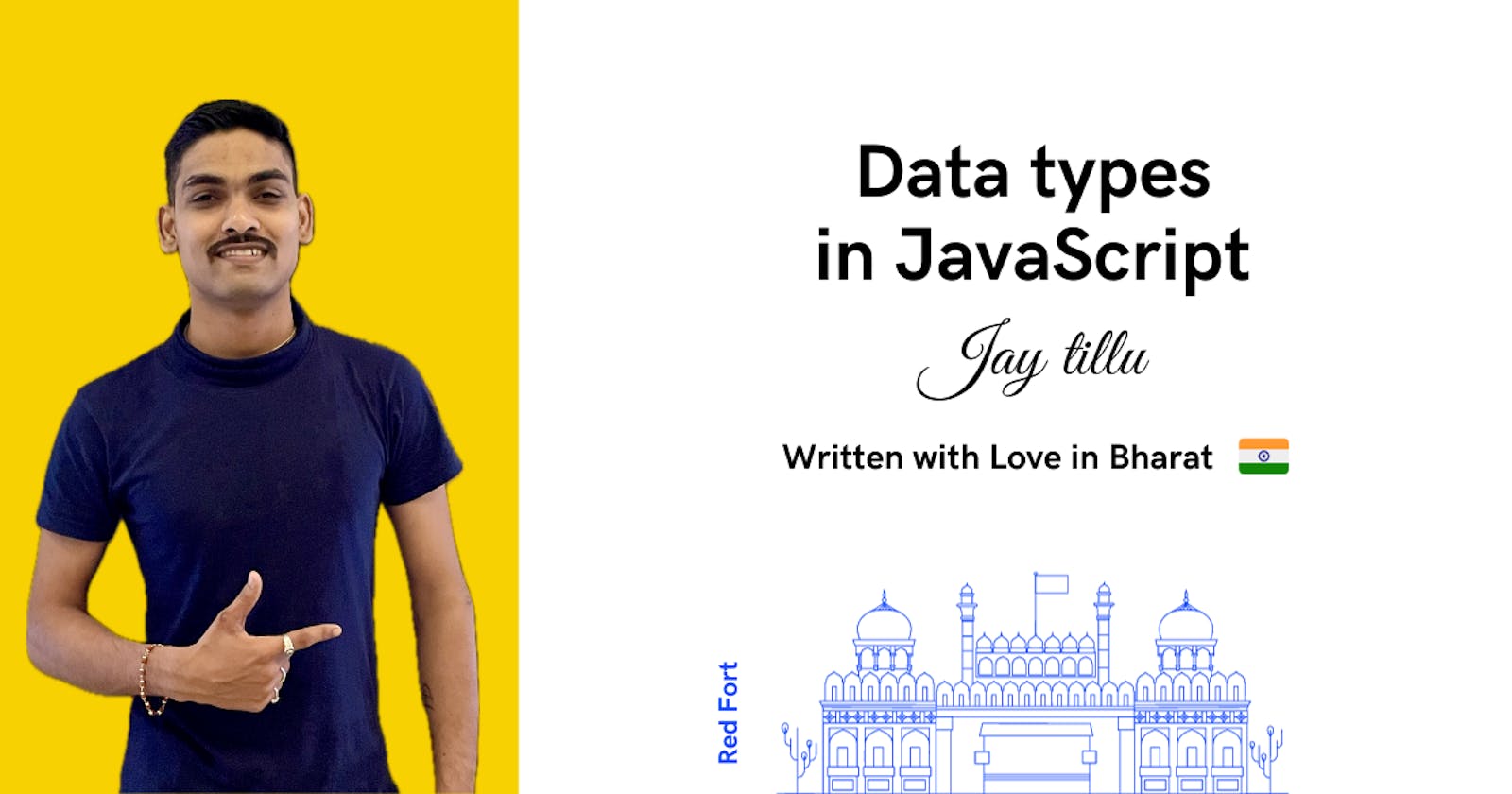
Datatypes are the foundational topic of any programming language. Today we will learn about Datatypes in JavaScript. Datatype specifies which type of data a language can store and process.
There are two types of Datatypes in JavaScript:
Primitive
Non-Primitive (Reference Types)
This categorization is made based on two parameters:
How we store value in memory
How we access the value from memory
Primitive Datatypes in JavaScript
Primitive types are Pass by Value. This means that copies of their value are used when passed to functions or assigned to other variables.
This behaviour is called Pass by Value because you are passing a copy of the value to the function. Primitive types (e.g., numbers, strings, booleans) are immutable in JavaScript, this means their values cannot be changed after creation. These values are like locked boxes โ you can't change what's inside. If you modify the value inside a function, it won't change the original value outside the function because you're working with a separate copy. When you modify a primitive type inside a function, you're working with a new copy of the value, leaving the original value unaffected.
There are seven primitive datatypes are there in JavaScript.
Number: Represents numeric values, both integers and floating-point numbers.
String: Represents textual data enclosed in single or double quotes.
Boolean: Represents a true or false value.
Undefined: Represents a variable that has been declared but hasn't been assigned a value.
Null: Represents an intentional absence of any value or object.
Symbol (introduced in ECMAScript 6): Represents a unique and immutable value, often used as object property keys.
BigInt (introduced in ECMAScript 11): Represents large integers that cannot be represented as regular JavaScript numbers.
Non-Primitive Datatypes in JavaScript
In JavaScript, Non-Primitive types are Passed by Sharing a Reference. This means changes made to copies will affect the original value since they share the same reference.
This means when you pass an object or an array into a function, you're passing a reference to the same object or array in memory. If you modify the object or array inside the function, those changes will affect the original object or array outside the function because you're working with the same underlying data.
It's important to understand that while it may seem like pass by reference, JavaScript is technically using pass by sharing a reference for these non-primitive types. This is a subtle but important distinction because you're not passing the actual object; you're sharing a reference to the object's location in memory.
There are mainly three Non-Primitive Datatypes in JavaScript:
Object: Represents complex data structures and collections of key-value pairs. Objects can be created with curly braces
{}
or with constructors likenew Object()
.Array: A special type of object that represents a list of values, indexed by integers, and is created using square brackets
[]
.Function: A type that represents executable code and is used for defining reusable functions. Functions are first-class citizens in JavaScript.
Date: Represents date and time information.
RegExp: Represents regular expressions used for pattern matching within strings.
Error: Represents errors thrown during runtime.
Return Type of Variables in JavaScript
Below are the Return Types of Every Variables in JavaScript. Remember them as they will help you in Interviews and in Programming as well. You can check the Return Type of Variables using typeof
operator. Below is a small example:
let num = 42;
let str = "Hello";
let obj = { key: "value" };
console.log(typeof num); // "number"
console.log(typeof str); // "string"
console.log(typeof obj); // "object"
Primitive Datatypes
Number -> number
String -> string
Boolean -> boolean
null -> object
undefined -> undefined
Symbol -> symbol
BigInt -> bigint
Non-Primitive Datatypes
Array -> object
Function -> function
Object -> object
Conclusion
JavaScript has two main categories of datatypes: Primitive and Non-Primitive (Reference Types).
Primitive Datatypes:
Number: For numeric values, including integers and floating-point numbers.
String: For textual data enclosed in single or double quotes.
Boolean: For true or false values.
Undefined: For declared but unassigned variables.
Null: For representing the intentional absence of a value or object.
Symbol (introduced in ECMAScript 6): Represents unique and immutable values, often used as object property keys.
BigInt (introduced in ECMAScript 11): Handles large integers that can't be represented as regular JavaScript numbers.
Primitive datatypes are passed by value, making copies of their values, and are immutable.
Non-Primitive Datatypes:
Object: Used for complex data structures and collections of key-value pairs. Created with curly braces or constructors.
Array: A special type of object representing lists of values, indexed by integers, created using square brackets.
Function: Represents executable code and defines reusable functions. Functions are first-class citizens in JavaScript.
Date: Handles date and time information.
RegExp: Manages regular expressions used for pattern matching within strings.
Error: Represents errors thrown during runtime.
Non-primitive datatypes are passed by sharing a reference, meaning changes made to copies will affect the original value, as they share the same reference to the object's location in memory.