JavaScript Pass by Value and Pass by Reference - Simplified
2 min read
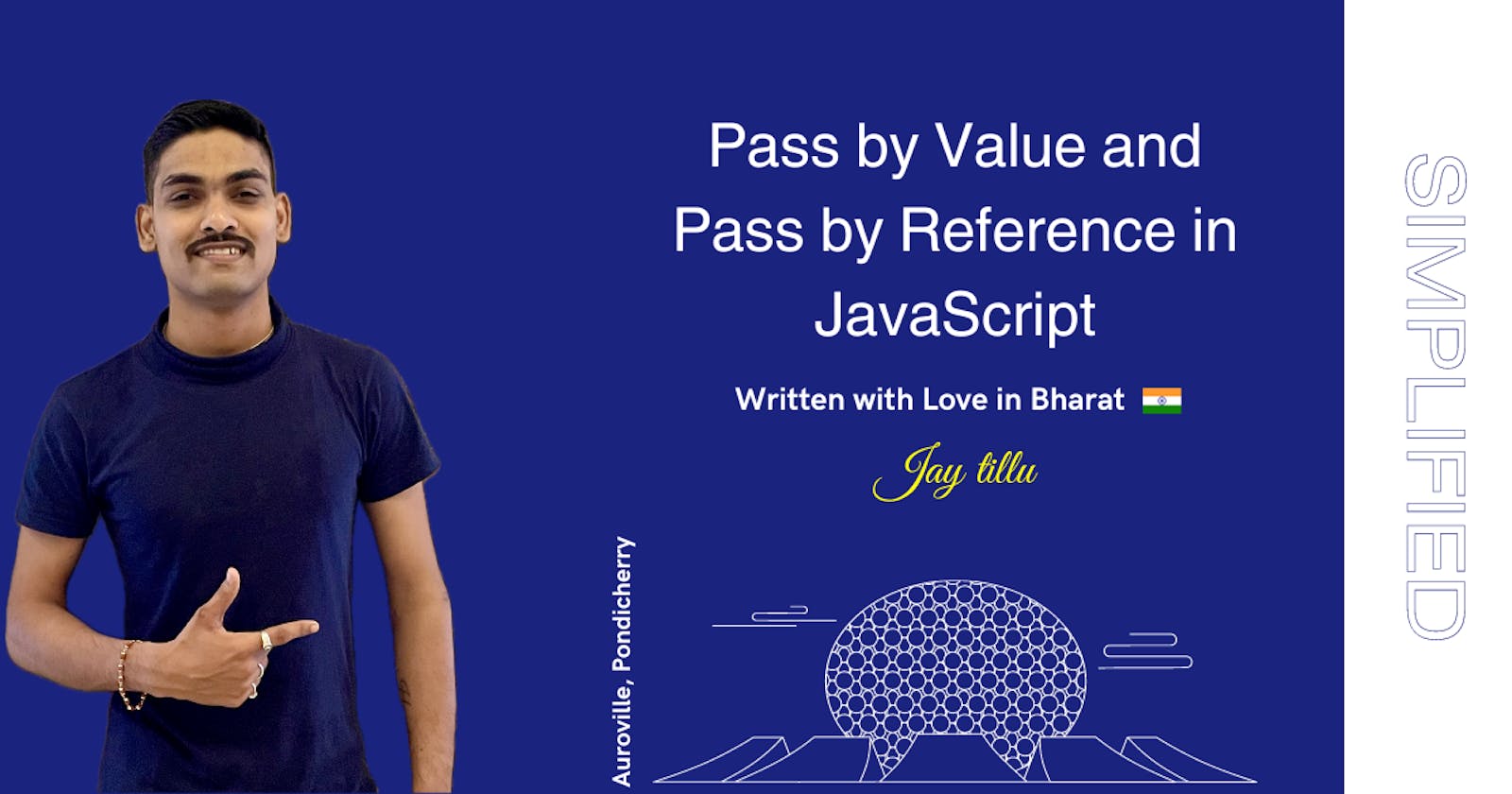
Based upon Datatype, JavaScript passes a value of a variable using two ways:
Using Pass by Value
Using Pass by Reference
Pass by Value
When you pass primitive data types (such as numbers, strings, or booleans) to a function, a copy of the actual value is passed. Changes made to that variable inside the function do not affect the original variable outside the function. These values are like locked boxes – you can't change what's inside. If you modify the value inside a function, it won't change the original value outside the function because you're working with a separate copy.
In simple terms, Pass by Value is like making a photocopy of a document: changing the copy doesn't affect the original document.
function modifyValue(x) {
x = 10;
}
let num = 5;
modifyValue(num);
console.log(num); // Output: 5
In the example above, the value num
remains unchanged because it's a primitive type.
Pass by Reference
When you pass an object (like an array or custom object) to a function, a reference to that object is sent to the function. Changes made inside the function affect the original object outside the function.
When you work with non-primitive types like objects or arrays, they are passed in a way that they share the same underlying data. Imagine it like two people holding a balloon. If one person changes the balloon's colour, the other person sees the change because they both have a grip on the same balloon. Similarly, if you modify an object or array inside a function, those changes are reflected outside the function because they both refer to the same object or array in memory.
function modifyObject(obj) {
obj.prop = "new value";
}
let myObject = { prop: "old value" };
modifyObject(myObject);
console.log(myObject.prop); // Output: "new value"
In this case, the object myObject
is passed by reference, so changes made to its property prop
inside the function are reflected in the original object.